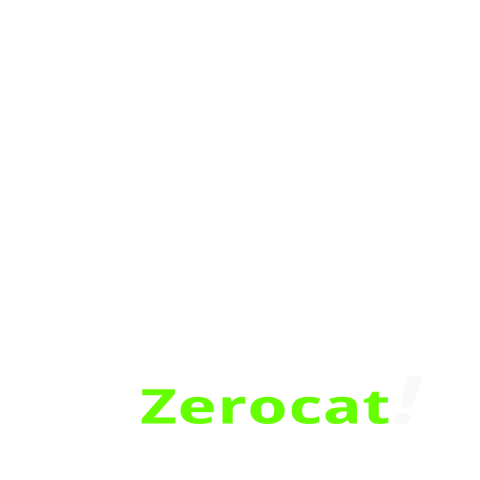 |
Zerocat Chipflasher
v0.4.3 (board-edition-1)
Flash free firmware to BIOS chips, kick the Manageability Engine.
|
Go to the documentation of this file.
27 #ifndef __CHIPSPEC_H__
28 #error "chipspec.h has not been included, yet."
31 #ifndef __SERIAL_CODES_H__
32 #error "serial-codes.h has not been included, yet."
35 #ifndef __FILESPEC_H__
36 #error "filespec.h has not been included, yet."
60 #define ERROR_MSG__LINE_TOO_LONG
65 #define HEADER_KICK " Zerocat Chipflasher: kick " VERSION " "
66 #define SIZE_STR_MESSAGE (1 << 7)
67 #define SIZE_STRBUF (1 << 8)
68 #define SIZE_LINEBUF (1 << 8)
69 #define BAUDRATE_DEFAULT 115200
70 #define NOT_APPLICABLE -1
71 #define POWERUP_SPI 25
72 #define POWERUP_SPILINES 25
73 #define POWERUP_EXTRA 2
74 #define POWERUP_OFFTIME 100000
75 #define STACK_DEFAULT 0x40
76 #define STACK_BURNBUF 0x20
77 #define STACK_LEDSTAT 0x20
78 #define MAX_POLLING 100000000
81 #define MODE_TERMINAL 0x01
82 #define MODE_CONNECT 0x02
85 #define MODE_SPI_OFF 0x00
86 #define MODE_FORCE_SPI_OFF 0x01
87 #define MODE_SPI_POWEROFF 0x02
90 #define LOOP_RES ((CLKFREQ / 1000000) * 1000) //1ms
91 #define STATUS_CYCLE 1000 //1000ms
92 #define LED_PHASE 90 //90ms
95 #define PUTCHAR_CR do { \
99 #define PUTCHAR_NL do { \
103 #define GET_CHAR_GROUP(n) for(unsigned char i = 0; i < n; i++) { \
104 c[i] = outfifo(getChar()); \
107 #define MSG_DONE "...done.\r\n\n"
109 #define SPI_ON SPI_ini()
110 #define SPI_OFF(mode) SPI_off(mode)
263 unsigned int * lines,
273 unsigned char addrlen_in_bits,
274 unsigned int firstloc,
275 unsigned int memsize,
276 unsigned char screenmode
302 const unsigned int value,
323 unsigned int firstloc,
324 unsigned int memsize,
325 unsigned int * lines,
348 unsigned char new_index
357 unsigned char odd_tracker
361 unsigned int firstloc,
365 inline unsigned char inb (
386 unsigned char level_rst
411 const unsigned char cmd,
412 const unsigned int value,
421 const unsigned char regno,
422 const unsigned char byte
442 unsigned char pswitch
451 const unsigned char blockcmd
459 unsigned char * key_is_valid,
468 const unsigned int addr,
469 unsigned char * buffer
void key_config(void)
Enable menu keys according to chip specs.
char MOT_rxline(struct tag_xcog0 *px, unsigned int *lines, int hexmode)
Receive and parse a line in Motorola S-Record format. This func finishes successfully after having pa...
int do__WIP_polling(void)
Invoking WIP_polling() usually is accompanied by some checks and messages.
void menu_options(void)
Put menu options on terminal screen.
void global_sector_protect(unsigned char pswitch)
Method to globally protect/unprotect all sectors.
unsigned int startaddr
Line’s startaddress.
volatile unsigned char queue_empty
Flag to indicate that the data queue is empty.
void PGM_cycle(enum tag_cmdstat *cmdstat, unsigned char odd_tracker)
unsigned char yes_no(char *question, unsigned char level_rst)
This function allows to put a question which expects true or false.
Struct that characterizes a chip.
signed char chip_read(struct tag_filespec *filespec, unsigned int firstloc, unsigned int memsize, unsigned int *lines, char *linebuf)
unsigned char abits
addrbits.
volatile unsigned char yellow
Switch for yellow LED usage.
void option_batchblockerase(const enum tag_blocksize blocksize, const unsigned char blockcmd)
Versatile block erase function, which allows erasing of multiple blocks of different sizes,...
unsigned int rd
Read pointer.
int hex2bin(unsigned char a, unsigned char b)
Converts a pair of hexadecimal chars into binary value.
volatile unsigned char green
Switch for green LED usage.
#define SIZE_32K
Size of 32K-block.
unsigned char get_addrlen(enum tag_type_of_addrlen type_of_addrlen, unsigned int addr)
Auxiliary function that returns the length of an address for different situations.
void MOT_summary(unsigned int lines, char *linebuf)
Struct that characterizes a data line.
void cmd_WREN(void)
Set Write Enable Latch bit.
unsigned char cmd
Command to be used for writing.
void page_write(const unsigned int addr, unsigned char *buffer)
Write a page buffer to chip.
void exit_sequence(const char status)
Tell terminal to exit listening mode.
volatile unsigned char rq_spioff
Flag to request SPI off.
void ledstat()
This function controls board LEDs. To be used with extra cog, to be stopped externally.
Motorola S-Record Type Infos.
void MOT_typeS_fillup(union tag_typeS *typeS)
Generating and parsing a Motorola S-record line needs to have some specs available about the line in ...
void cmd_WRSR(const unsigned char regno, const unsigned char byte)
Write to Status Registers.
unsigned char cmd_RDSR(unsigned char regno)
Read the status register.
unsigned int lineaddr
startaddress of data line.
void chip_ini(unsigned char new_index)
int strpos(char *str, char c)
Report the position of a character in a string.
unsigned int inbits(unsigned int msbit)
unsigned int CPM_polling(void)
Poll CPM bit.
void check_dryrun(void)
Checks wether a write fail should be expected. A function with very basic and poor functionality.
void chip_erase(void)
This function calls the global chip erase command.
void MOT_header(char *header, char *linebuf)
This function invokes MOT_mkline(), but initializes parameters first with apropriate header data.
char HEXDUMP_rxline(struct tag_xcog0 *px, unsigned int *lines)
Parse a Hexdump line.
volatile unsigned char orange
Switch for orange LED usage.
volatile unsigned int offrd
Buffer read pointer offset.
void MOT_mkline(struct tag_linedat *linedat, union tag_typeS *typeS, char *linebuf)
This function wraps a binary payload into a Motorola S-record frame.
volatile unsigned char rq_lineaddr
Flag to request the next line address.
void menu_line(unsigned int k1, char *k1msg, unsigned int k2, char *k2msg, unsigned int k3, char *k3msg)
Put a menu line with selected menu options on terminal screen.
unsigned char * pbuf
Pointer to ringbuffer.
#define SIZE_64K
Size of 64K-block.
void key_polling(unsigned char *key_is_valid, unsigned char *quit)
Essential function. Scans stdin for configured keys and issues the apropriate actions....
unsigned int wr
Write pointer.
void SR_intro(unsigned char regno)
Provide each bit listing of an status register with a short header.
void chip_txfile(struct tag_filespec *filespec, unsigned char addrlen_in_bits, unsigned int firstloc, unsigned int memsize, unsigned char screenmode)
int plug_detect(void)
Check if an SPI-Plug is detected and put a message on screen.
void report_err(char reported_err)
void range_error(void)
Reports an Out-of-Range user input error and resets xcog1.inlevel.
void chip_rxfile(struct tag_xcog0 *px, char screen_output)
void burn()
Wite data to chip.
struct tag_filespec filespec[2]
unsigned char SR_report(unsigned char regno)
Put status register's content on screen.
void outbits(const unsigned int value, unsigned int msbit)
Cog parameters for ledstat().
int pages
Number of pages (on a paged chip).
unsigned char verify_0xff(unsigned int firstloc, unsigned int memsize)
This function scans the specified chip memory for values different than 0xff. If a value different to...
struct tag_chipspec * pspec
Pointer into chipspec database.
#define SIZE_4K
Size of 4K-sector.
void SPI_ini(void)
Initialize SPI bus; activate hardware write protection.
unsigned char addrlen_in_bits
Number of bits that are used feed an address into the SPI-bus.
void MOT_data(struct tag_linedat *linedat, char *linebuf)
volatile unsigned char inlevel
Current menu input level.
volatile unsigned int offwr
Buffer write pointer offset.
void option_readblock(const enum tag_blocksize blocksize)
void SPI_off(int mode)
Switch SPI bus off.
void mirror(char *keymsg)
int WIP_polling(void)
Determine end of write cycle by polling the WIP bit.
unsigned int mask
Mask for read and write pointers.
void menu(unsigned char mode)
Put the chipflasher menu on screen and invoke key_polling().
unsigned char bufsize
Size of ringbuffer.
signed char linebuf_out(char *linebuf)
Put a formatted data line onto tty.
Cog parameters for burn().
int * pcog
Cog info pointer, used by cog_end().
char * buf
Pointer to buffer, that holds the data.
void cmd(const unsigned char cmd, const unsigned int value, unsigned char bits)
Chip specifications, provided within the source code.
unsigned char payload
Number of payload bytes.
char queue(struct tag_xcog0 *px, unsigned char vbin, unsigned int *pwr)
void HEXDUMP_mkline(struct tag_linedat *linedat, char *linebuf_orig)
This function transforms a binary payload into a row of ascii chars including line ending characters ...
void greeting()
Put a small headline on terminal screen that helps to identify the program.
void hr(char c, int n, char nl)
Put a horizontal line on screen.