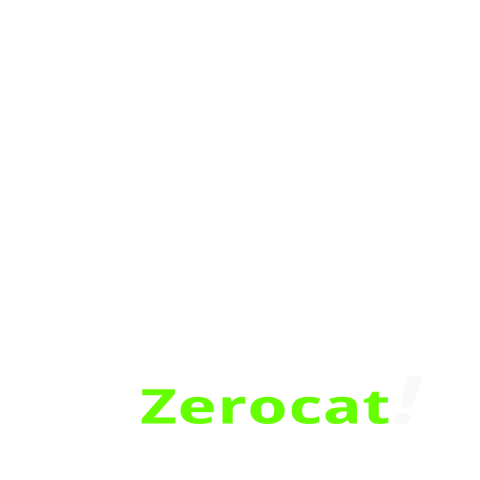 |
Zerocat Chipflasher
v0.4.3 (board-edition-1)
Flash free firmware to BIOS chips, kick the Manageability Engine.
|
Go to the documentation of this file.
27 #ifndef __CHIPSPEC_H__
28 #define __CHIPSPEC_H__
69 #define ARRAY_CHIPSPEC 13
70 #define CHIPSPEC_DEFAULT 0
79 #define SIZE_1 0x00000001
80 #define SIZE_2 0x00000002
81 #define SIZE_4 0x00000004
82 #define SIZE_8 0x00000008
83 #define SIZE_16 0x00000010
84 #define SIZE_32 0x00000020
85 #define SIZE_64 0x00000040
86 #define SIZE_256 0x00000100
87 #define SIZE_1K 0x00000400
88 #define SIZE_4K 0x00001000
89 #define SIZE_16K 0x00004000
90 #define SIZE_32K 0x00008000
91 #define SIZE_64K 0x00010000
92 #define SIZE_4MBIT 0x00080000
93 #define SIZE_8MBIT 0x00100000
94 #define SIZE_16MBIT 0x00200000
95 #define SIZE_32MBIT 0x00400000
96 #define SIZE_64MBIT 0x00800000
97 #define SIZE_128MBIT 0x01000000
98 #define SIZE_256MBIT 0x02000000
107 #define ID_JEDEC_AT26DF321 0x001f4700
108 #define ID_JEDEC_AT26DF161 0x001f4600
121 #define X20_SE 2 //Sector Erase (4K)
122 #define X52_BE32K 3 //Block Erase (32K)
123 #define XD8_BE64K 4 //Block Erase (64K)
124 #define X60_CE 5 //Full Chip Erase
125 #define XC7_CE 6 //Full Chip Erase
126 #define X02_PP 7 //Page Program
127 #define XAD_CP 8 //Continuous Program
128 #define X02_BP 9 //Single Byte Program
130 #define X06_WREN 10 //Write Enable
131 #define X04_WRDI 11 //Write Disable
132 #define X50_EWSR 12 //Enable Write Status Register
134 #define X05_RDSR 13 //Read Status Register
135 #define X01_WRSR 14 //Write Status Register
138 #define X00_NOP 15 //if this is set, a NOP is send to the device after X9F_RDID.
140 #define X9F_RDID 16 //Read JEDEC Manufacturer and Device ID (3 Databytes)
141 #define XB9_DP 17 //Enter Deep Powerdown
142 #define XAB_RDP 18 //Release from Deep Powerdown
144 #define XAB_RES 19 //Read Electronic Signature (1 Databyte)
145 #define X90_REMS 20 //Read Manufacturer and Device ID (2 Databytes)
146 #define X3C_RSPR 21 //Read Sector Protection Registers
147 #define X36_PS 22 //Protect Sector Command (Block 4K)
148 #define X39_US 23 //Unprotect Sector Command (Block 4K)
149 #define X70_ESRY 24 //Enable Ready at Pin
150 #define X80_DSRY 25 //Disable Ready at Pin
151 #define X35_RDSR2 26 //Read Status Register 2
152 #define X15_RDSR3 27 //Read Status Register 3
153 #define X31_WRSR2 28 //Write Status Register 2
154 #define X11_WRSR3 29 //Write Status Register 3
char name[15]
chip's name, i.e. MX25L1605D
const unsigned int chipsize
Size of the chip.
const int pagesize
If not applicable or unknown, clear cmdset.X02_PP.
const unsigned char sr_is_static[3]
True, if Status Register is not initialized during Power-Up.
const int cmdset
selected commands
const signed char sizeOTP
If not applicable or unknown, clear cmdset.X2B_RDSCUR.
const int id_JEDEC
If not applicable or unknown, clear cmdset.X9F_RDID.
const unsigned char sr_wrmask[3]
maskout unwritable bits of the status register
Chip specifications, provided within the source code.
char * sr_bitnames[3]
Status Register 0 Bit Names.